Mastering OOP, OOAD, and SOLID Principles for Interview Success
Understanding OOP, OOAD, and SOLID Principles for Interview Preparation
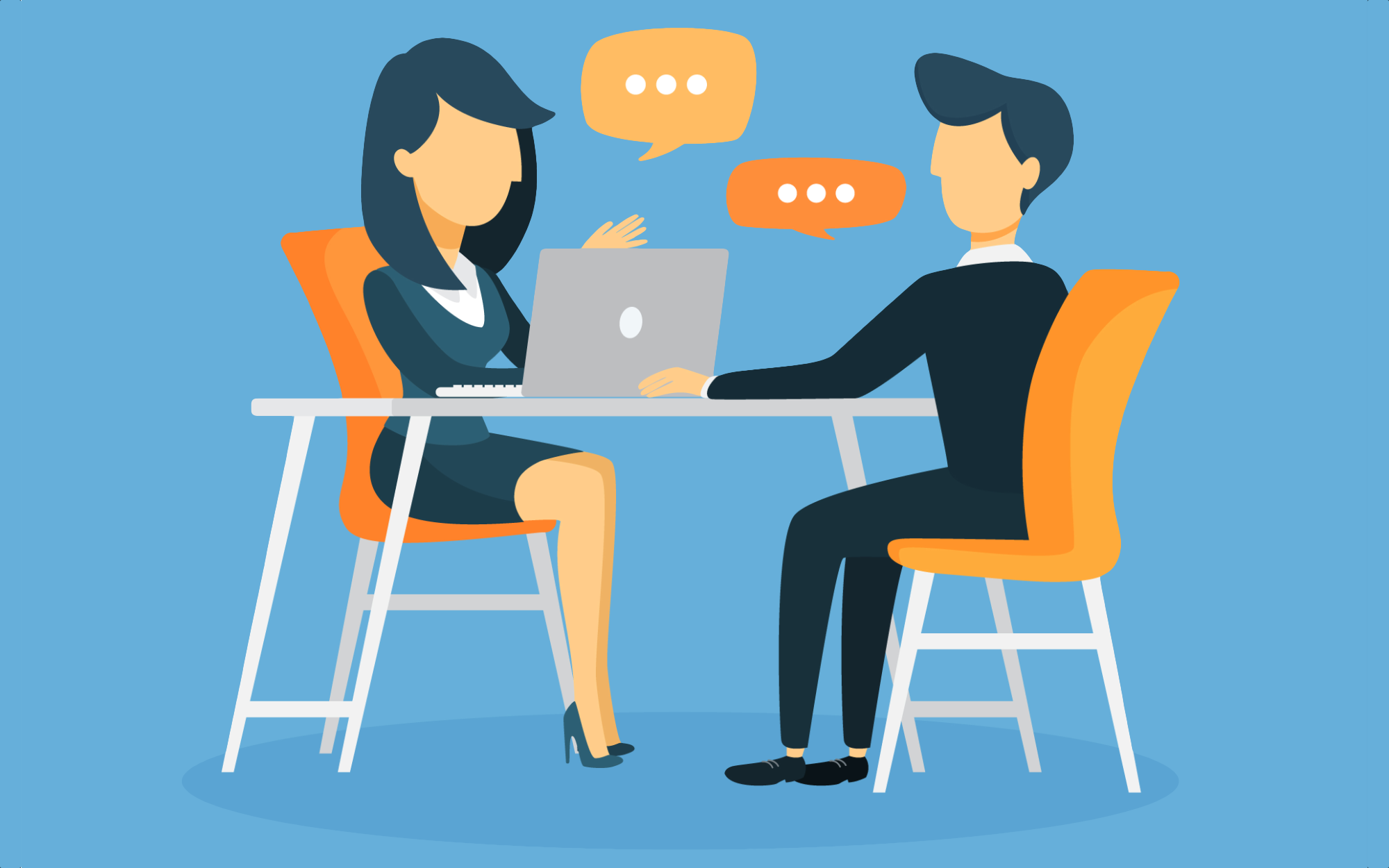
Object-oriented concepts are a core part of software development, especially in the context of interviews for software engineering and quality assurance roles. In this post, we’ll go through the fundamentals of Object-Oriented Programming (OOP), Object-Oriented Analysis and Design (OOAD), and SOLID principles with examples to help you prepare for technical interviews.
What is OOP?
Object-Oriented Programming (OOP) is a programming paradigm that organizes code around objects rather than actions. Unlike procedural programming, which focuses on functions, OOP focuses on creating reusable structures, making it easier to manage large projects. OOP lets you group related data and functionality into classes, which can be thought of as blueprints for creating individual objects.
Key Concepts of OOP
To understand OOP, let’s explore its four main principles: Encapsulation, Inheritance, Polymorphism, and Abstraction. Each of these helps you organize and structure code in a way that is modular, scalable, and easier to maintain.
1. Encapsulation
Encapsulation is the concept of bundling data (variables) and methods (functions) together within a single unit, known as a class. It allows you to restrict access to certain details of an object and expose only what is necessary. By keeping some data private, you protect it from unintended changes.
Example:
Imagine a Car
class where each car has a color
and brand
. The color
is kept private to prevent direct changes from outside the class, but there are get_color
and set_color
methods to control access.
class Car:
def __init__(self, color, brand):
self.__color = color # private variable
self.__brand = brand # brand can be accessed directly
def get_color(self):
return self.__color
def set_color(self, color):
self.__color = color
In this example: __color
is a private variable. Using two underscores before the variable name makes it private. The get_color
method retrieves the color, while set_color
allows us to change it. This way, we control how color is accessed and modified.
Why Encapsulation?
Encapsulation ensures that:
- Sensitive data is hidden from the outside.
- Changes to data only occur in controlled ways, helping avoid unintended errors.
- Inheritance
Inheritance allows a class to inherit properties and behaviors (methods) from another class. This enables code reuse and establishes a hierarchical relationship between classes. Think of inheritance as a way to create specialized versions of existing classes.
Example:
Let’s expand our Vehicle example by creating a Car class that inherits from Vehicle.
class Vehicle:
def __init__(self, brand):
self.brand = brand
def honk(self):
return "Beep Beep!"
class Car(Vehicle):
def __init__(self, brand, model):
super().__init__(brand) # inherit brand from Vehicle
self.model = model
my_car = Car("Toyota", "Corolla")
print(my_car.honk()) # Output: Beep Beep!
Here: • Car is a subclass of Vehicle. • Car inherits the honk method from Vehicle, so Car objects can call it. • Using super(), we initialize the brand attribute from the parent class, Vehicle.
Why Inheritance?
Inheritance helps: • Avoid code duplication, as common properties can be placed in a parent class. • Establish relationships between classes, creating a clearer, more organized code structure.
- Polymorphism
Polymorphism allows you to use methods from different classes in a similar way, even if they behave differently. This enables you to write code that works with objects of different classes interchangeably, as long as they share common methods.
Example:
Let’s create Dog and Cat classes, each with a sound method, and demonstrate how we can call sound on different types of animals.
class Dog:
def sound(self):
return "Woof!"
class Cat:
def sound(self):
return "Meow!"
def animal_sound(animal):
return animal.sound()
print(animal_sound(Dog())) # Output: Woof!
print(animal_sound(Cat())) # Output: Meow!
In this example: • Both Dog and Cat have a sound method. • The animal_sound function takes an animal and calls sound, regardless of whether it’s a dog or a cat.
Why Polymorphism?
Polymorphism allows: • Flexibility, as you can use the same interface for different data types. • Simplified code, as common methods can be reused across various classes.
- Abstraction
Abstraction focuses on hiding complex details and showing only the essential features of an object. In OOP, abstraction is often achieved using abstract classes or interfaces. Abstract classes act as templates that define what methods subclasses must implement without specifying the exact implementation.
Example:
Let’s create an abstract Animal class with an abstract method sound, and subclasses Dog and Cat that define how each animal makes a sound.
from abc import ABC, abstractmethod
class Animal(ABC): # abstract base class
@abstractmethod
def sound(self):
pass
class Dog(Animal):
def sound(self):
return "Woof!"
class Cat(Animal):
def sound(self):
return "Meow!"
Here: • Animal is an abstract class that defines a template method sound. • Dog and Cat are subclasses that implement the sound method with specific behaviors.
Why Abstraction?
Abstraction helps: • Simplify complex systems by exposing only the necessary parts. • Enforce certain behaviors in subclasses, ensuring consistency across your code.
Now let’s talk about OOAD:
OOAD is a structured approach to software design that combines object-oriented programming principles with a method for analyzing and designing applications. This approach focuses on creating a blueprint for solving a specific problem, which includes: 1. Identifying requirements: Analyze the needs and goals of the application. 2. Defining classes: Determine the main classes that will be used. 3. Designing interactions: Define relationships, associations, and inheritance between classes.
OOAD Example
Suppose we’re designing a simple e-commerce application. Here’s an OOAD approach: 1. Identify requirements: We need Product, Customer, and Order classes. 2. Define relationships: A Customer can place multiple Orders, and an Order can contain multiple Products. 3. Define interactions: Methods for add_product, checkout, etc., can be designed to encapsulate functionalities in relevant classes.
SOLID Principles
SOLID principles are five design principles that help make software designs more understandable, flexible, and maintainable. Here’s a breakdown of each principle: 1. Single Responsibility Principle (SRP) A class should have only one reason to change, meaning it should only have one job or responsibility. Example:
class EmailService:
def send_email(self, to, subject, body):
# Sends email logic here
pass
- Open/Closed Principle (OCP) Classes should be open for extension but closed for modification. This means we should be able to add new functionality without changing existing code. Example:
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Rectangle(Shape):
def area(self):
return self.width * self.height
-
Liskov Substitution Principle (LSP) Objects of a superclass should be replaceable with objects of a subclass without affecting the functionality of the application.
-
Interface Segregation Principle (ISP) Clients should not be forced to implement interfaces they don’t use. Split interfaces into smaller, more specific ones. Example: ```python class PrintInterface(ABC): @abstractmethod def print(self): pass
class ScanInterface(ABC): @abstractmethod def scan(self): pass ```
Interview Preparation Tips
Understanding and explaining these principles can be crucial in interviews. Here are some tips to prepare: • Understand core concepts: Focus on understanding OOP and SOLID principles thoroughly rather than memorizing definitions. • Prepare examples: Practice writing simple examples for each concept. Coding platforms like LeetCode and HackerRank can provide practical scenarios to apply these principles. • Mock Interviews: Practice explaining these concepts in mock interviews, focusing on real-world scenarios or projects you have worked on. • Practice Design Patterns: Some interviewers may ask about design patterns, which are built upon SOLID principles and can be helpful in showing your knowledge of maintainable code.
By mastering OOP, OOAD, and SOLID principles, you’ll be better prepared to solve real-world problems, showcase your understanding in interviews, and create more robust and maintainable code. Good luck with your interview preparation!
Happy coding!
Enjoy Reading This Article?
Here are some more articles you might like to read next: